In this post we are going to go over the Instagram Graph API PHP SDK! I created this SDK because interacting with the Instagram Graph API can be frustrating and confusing at times and I wanted to make it easier to make API calls and interact with the Instagram Graph API. This is an open source SDK aimed at making the Instagram Graph API easier to learn and use!
SDK Code Setup and Structure
The GitHub Repository is setup for the most part to mimic the Instagram Graph API Documentation. Each reference section in the documentation maps to a folder in the SDK.
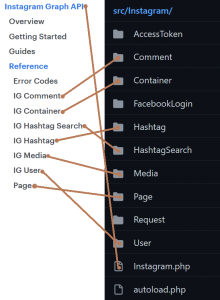
Then inside each of those folders is a class for each endpoint. Inside each folder there is also a main class which all the other classes in the folder extent. The main classes in each folder also extend the master Instagram.php class which is in the root folder.
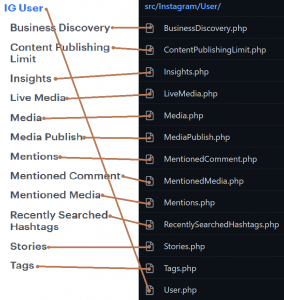
Installation
To install the SDK you can opt for the Composer route, or you can simply download the code from the github repo. Here are examples of both.
Composer
Run this command:
composer require jstolpe/instagram-graph-api-php-sdk
Require the autoloader.
require_once __DIR__ . '/vendor/autoload.php'; // change path as needed
GitHub
git clone git@github.com:jstolpe/instagram-graph-api-php-sdk.git
Require the custom autoloader.
require_once '/instagram-graph-api-php-sdk/src/instagram/autoload.php'; // change path as needed
GET Request
Here is an example of a simple GET request for getting a user’s profile and media posts. All we have to do is pass along the Instagram User ID, username, and an access token.
use Instagram\User\BusinessDiscovery; $config = array( // instantiation config params 'user_id' => 'IG_USER_ID', 'username' => 'USERNAME', // string of the Instagram account username to get data on 'access_token' => 'ACCESS_TOKEN', ); // instantiate business discovery for a user $businessDiscovery = new BusinessDiscovery( $config ); // initial business discovery $userBusinessDiscovery = $businessDiscovery->getSelf();
POST Request
Here is simple POST example of posting an image to an Instagram account. The variables we need for the config are the Instagram User ID and an access token. Then, when we are creating the image container we need to specify the caption for the post, and then a url to the image and the image must be on a public server.
use Instagram\User\Media; use Instagram\User\MediaPublish; $config = array( // instantiation config params 'user_id' => 'USER_ID', 'access_token' => 'ACCESS_TOKEN', ); // instantiate user media $media = new Media( $config ); $imageContainerParams = array( // container parameters for the image post 'caption' => 'CAPTION', // caption for the post 'image_url' => 'IMAGE_URL', // url to the image must be on a public server ); // create image container $imageContainer = $media->create( $imageContainerParams ); // get id of the image container $imageContainerId = $imageContainer['id']; // instantiate media publish $mediaPublish = new MediaPublish( $config ); // post our container with its contents to instagram $publishedPost = $mediaPublish->create( $imageContainerId );
CUSTOM Request
Here is an example of a custom request. The SDK allows you to fully specify the request type, endpoint, and parameters for the endpoint. Like all requests we first need an access token. Then we are ready to make our request (get/post/delete). Specify the endpoint to hit along with the parameters for the endpoint. The parameters array is key/value paired where the key must match what the IG API is expecting according to the documentation and you can add as many or as little as you need.
// first we have to instantiate the core Instagram class with our access token $instagram = new Instagram\Instagram( array( 'access_token' => 'ACCESS_TOKEN' ) ); /** * Here we are making our request to instagram and specify the endpoint along with our custom params. * There is a custom function for get, post, and delete. * $instagram->get() * $instagram->post() * $instagram->delete() * * Here is the skeleton for the customized call. */ $response = $instagram->method( array( 'endpoint' => '/ENDPOINT', 'params' => array( // query params key/values must match what IG API is expecting for the endpoint 'KEY' => 'VALUE', 'KEY' => 'VALUE', // ... ) ) );
More Functionality
The Instagram Graph API PHP SDK covers and contains functionality for most of the Instagram Graph API endpoints. This post was a high level overview and in future blog posts I will cover specific endpoints in more detail. The GitHub Wiki contains documentation for the entire SDK with examples for how to call every endpoint.
Links
That is going to do it for this post! Leave any comments/questions below and thanks for stopping by the blog!